Il Timer in Swing
Ci sto lavorando
Classe molto utile che in swing ci permette di temporizzare qualsiasi azione.
Esempi: |
1 |

|
Mr.Webmaster - Creare una sveglia con timer |
2 |
 |
Contatore |
3 |
 |
|
4 |
 |
|
Procediamo con un esempio molto semplice:
Un Contatore: una finestra che mostra un numero che ogni mezzo secondo s'incrementa:
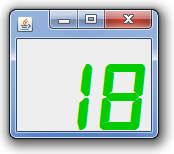
In modo tradizionale:
1 package mytimer;
2
3 import java.awt.event.*;
4 import javax.swing.*;
5
6 public class MyTimer {
7 int cnt=0;
8 public MyTimer(){
9 JFrame jf = new JFrame();
10 jf.setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
11 jf.setBounds(new java.awt.Rectangle(800, 500, 150, 130));
12 jf.setVisible(true);
13
14 JLabel jl = new JLabel();
15 jl.setFont(new java.awt.Font("Digital-7 Mono", 3, 100));
16 jl.setForeground(new java.awt.Color(0, 204, 0));
17 jl.setHorizontalAlignment(javax.swing.SwingConstants.RIGHT);
18 jl.setText("0");
19 jl.setBounds(0, 0, 270, 50);
20
21 jf.getContentPane().add(jl);
22
23 class TimerHandler implements ActionListener {
24 public void actionPerformed(ActionEvent e) {
25 cnt++;
26 jl.setText(""+cnt);
27 }
28 }
29 Timer t = new Timer(500,new TimerHandler());
30 t.start();
31 }
32
33 public static void main(String[] args) {
34 new MyTimer();
35 }
36 } |
Con una classe anonima:
4 import java.awt.event.*;
5 import javax.swing.*;
6
7 public class MyTimer {
8 int cnt=0;
9 public MyTimer(){
10 JFrame jf = new JFrame();
11 jf.setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
12 jf.setBounds(new java.awt.Rectangle(800, 500, 150, 130));
13 jf.setVisible(true);
14
15 JLabel jl = new JLabel();
16 jl.setFont(new java.awt.Font("Digital-7 Mono", 3, 100));
17 jl.setForeground(new java.awt.Color(0, 204, 0));
18 jl.setHorizontalAlignment(javax.swing.SwingConstants.RIGHT);
19 jl.setText("0");
20 jl.setBounds(0, 0, 270, 50);
21
22 jf.getContentPane().add(jl);
23
24 new Timer(500,new ActionListener() {
25 public void actionPerformed(ActionEvent evt) {
26 cnt++;
27 jl.setText(""+cnt);
28 }
29 }).start();
30
31 }
32
33 public static void main(String[] args) {
34 new MyTimer();
35 }
36 }
|
Discussione:
La classe Timer ha un costruttore: Timer(int delay, ActionListener listener)
il primo parametro da il tempo di attesa in millisecondi , il secondo è un acsoltatore che eseguirà qualcosa quando al termine dell'attesa verrà lanciato un evento. I metodi fondamentali sono:
setRepeats(false) - Se true (default) viene lanciato un evento al termine del tempo di attesa ripetutamente, se il delay è di un secondo ogni secondo partirà un evento. Se è false ci sarà una sola attesa ed un soo evento.
start() - parte e stop() - si ferma. Ce ne sono altri ma questi sono gli essenziali.
|