CenteredPanelExample
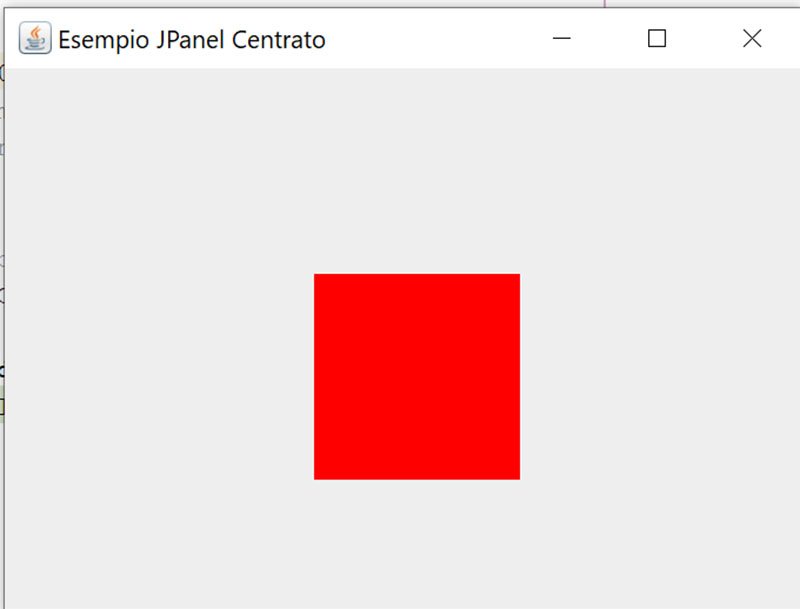
Classe: CenteredPanelExample - fig1 |

|
1 package centeredpanelexample;
2
3 import javax.swing.*;
4 import java.awt.*;
5 import java.awt.event.ComponentAdapter;
6 import java.awt.event.ComponentEvent;
7
8 public class CenteredPanelExample {
9 public static void main(String[] args) {
10 SwingUtilities.invokeLater(() -> {
11 JFrame frame = new JFrame("Esempio JPanel Centrato");
12 frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
13 frame.setSize(400, 300);
14 frame.setLocationRelativeTo(null);
15 frame.getContentPane().setLayout(null);
16
17 JPanel panel = new JPanel();
18 panel.setBackground(Color.RED);
19
20 panel.setBounds(50, 50, 100, 100);
21
22 frame.add(panel);
23
24
25 frame.addComponentListener(new ComponentAdapter() {
26 @Override
27 public void componentResized(ComponentEvent e) {
28 centerPanel(frame, panel);
29 }
30 });
31
32
33 frame.setVisible(true);
34 });
35 }
36
37
38 private static void centerPanel(JFrame frame, JPanel panel) {
39 int frameWidth = frame.getWidth();
40 int frameHeight = frame.getHeight();
41 int panelWidth = panel.getWidth();
42 int panelHeight = panel.getHeight();
43
44 int x = (frameWidth - panelWidth) / 2;
45 int y = (frameHeight - panelHeight) / 2;
46
47 panel.setLocation(x, y);
48 }
49 } |
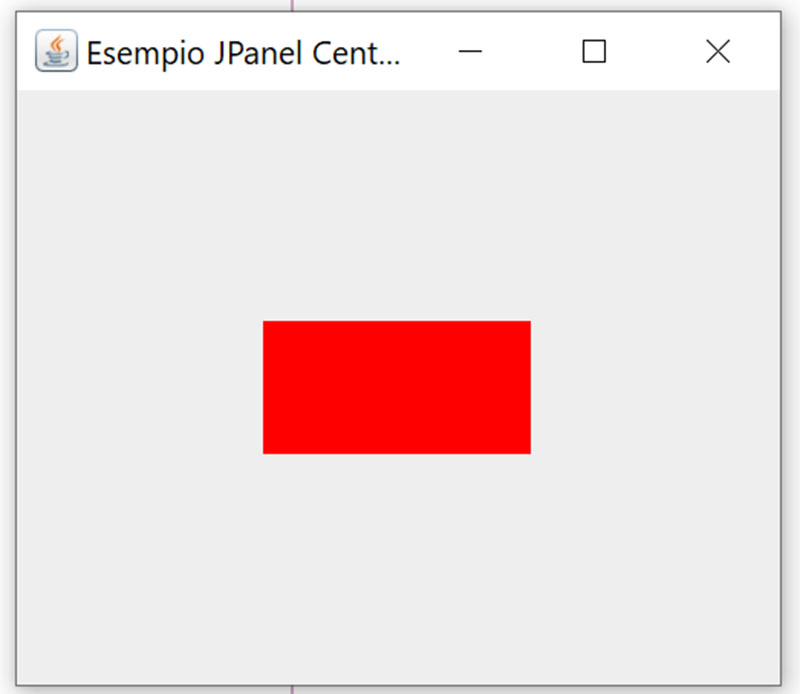
Classe: CenteredPanelExample - fig2 |

|
1 package centeredpanelexample;
2
3 import javax.swing.*;
4 import java.awt.*;
5
6 public class CenteredPanelExample {
7 public static void main(String[] args) {
8 SwingUtilities.invokeLater(() -> {
9 JFrame frame = new JFrame("Esempio JPanel Centrato");
10 frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
11 frame.setSize(400, 300);
12
13
14 JPanel panel = new JPanel();
15 panel.setBackground(Color.RED);
16 panel.setPreferredSize(new Dimension(100, 50));
17
18
19 frame.setLayout(new GridBagLayout());
20
21
22 GridBagConstraints constraints = new GridBagConstraints();
23 constraints.gridx = 0;
24 constraints.gridy = 0;
25 constraints.weightx = 1.0;
26 constraints.weighty = 1.0;
27 constraints.fill = GridBagConstraints.CENTER;
28 frame.add(panel, constraints);
29
30
31 frame.setVisible(true);
32 });
33 }
34 }
35 |
Ecco quale è il risultato se utilizziamo il codice che segue:
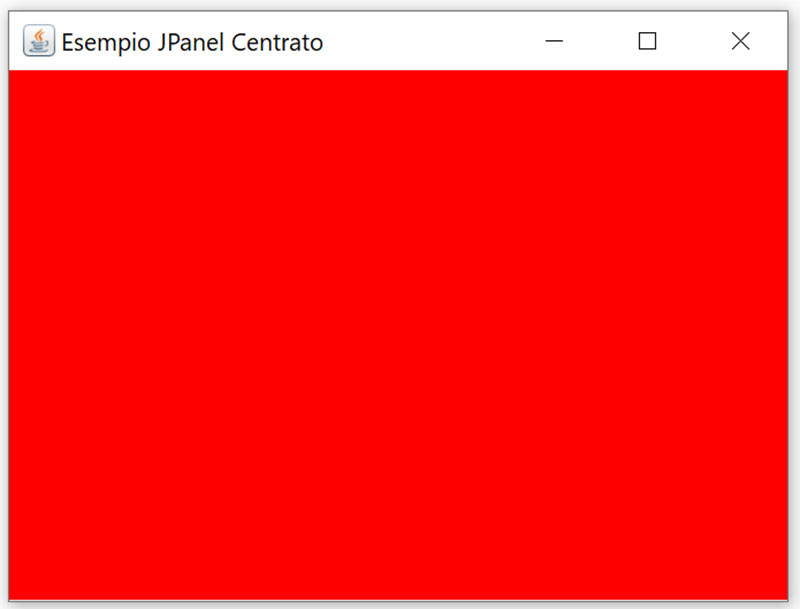
Classe: CenteredPanelExample - fig3 |

|
1 package centeredpanelexample;
2
3 import javax.swing.*;
4 import java.awt.*;
5
6 public class CenteredPanelExample {
7 public static void main(String[] args) {
8 SwingUtilities.invokeLater(() -> {
9 JFrame frame = new JFrame("Esempio JPanel Centrato");
10 frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
11 frame.setSize(400, 300);
12
13
14 JPanel panel = new JPanel();
15 panel.setBackground(Color.RED);
16 panel.setPreferredSize(new Dimension(100, 50));
17
18
19 frame.setLayout(new GridBagLayout());
20
21
22 GridBagConstraints constraints = new GridBagConstraints();
23 constraints.gridx = 0;
24 constraints.gridy = 0;
25 constraints.weightx = 1.0;
26 constraints.weighty = 1.0;
27
28 constraints.fill = GridBagConstraints.BOTH;
29 frame.add(panel, constraints);
30
31
32 frame.setVisible(true);
33 });
34 }
35 } |
In questi ultimi due codici c'è una differenza nel tipo di GridBagConstraints. nel primo è .CENTER mentre nl secondo è .BOTH. Nel primo il pannello viene compresso al centro ma rispetta le dimensioni impostae , mentre l'atro ignora le dimensioni assegnate ed espande il pannello sia in altezza che in larghezza fino ai bordi della finestra.
Risorse per approfondire l'argomento Layout:
Ecco alcune risorse che possono aiutarti a comprendere meglio i layout manager in Java:
-
A Visual Guide to Layout Managers:
- Questo tutorial di Oracle fornisce una panoramica visuale dei layout manager disponibili in Java Swing.
- Esamina ciascun layout manager (come BorderLayout, BoxLayout, CardLayout, FlowLayout, GridBagLayout, GroupLayout e SpringLayout) e offre esempi di GUI che utilizzano tali layout.
- È un buon punto di partenza per capire come funzionano i vari layout manager.
-
Lesson: Laying Out Components Within a Container:
- Questo tutorial di Oracle copre dettagliatamente come posizionare e dimensionare i componenti all’interno di un contenitore.
- Include informazioni su come impostare il layout manager, fornire suggerimenti di dimensioni e allineamento, gestire lo spazio tra i componenti e altro ancora.
-
How to Use Various Layout Managers:
- Questa pagina elenca i vari layout manager e ti indirizza alle pagine specifiche per ciascun tipo di layout.
- Puoi trovare esempi pratici e istruzioni dettagliate su come utilizzare ciascun layout manager.
-
Layout Manager in Java with Examples:
- Questo tutorial offre esempi pratici di come utilizzare i layout manager in Java.
- Copre i concetti di base e fornisce esempi di codice per ciascun tipo di layout manager.
-
Using Layout Managers:
- Questa pagina spiega come utilizzare i layout manager in modo più generale.
- Discute l’importanza dei layout manager e come influenzano la posizione e le dimensioni dei componenti all’interno di un contenitore.
|